MirChord
An overview of the MirChord notation language and a reference to the most common elements, symbols and commands. Learn how to write an entire multipart and multivoice score.
Comments
Everything that starts with ;
is ignored by the interpreter.
; This is a comment
Parts and Voices
A score is composed of one or more parts (up to 16 as in MIDI) and every part could have one or more voices.
A part graphically is rendered as a staff.
A part/staff is defined with the symbol = followed by an integer number.
Similarly a voice is defined with the symbol ~ followed by an integer number. Simple polyphony is managed that way.
So usually most scores start as follow: =1 ~1
. This means that we are in the first voice of the first part.
Warning: staff and voice definitions are required (not optional) in the score.
Key Signatures
A key signature can be defined using the key command,
Examples: to define a C major key you can write (key "C" "maj")
Tip: start writing something like key... and the autocomplete will propose all the available key signatures together with useful information like the number of sharps/flats.
Info: there are two different ways to define a key: specifing the fifths or mode.
Clefs
Clefs can be defined using the clef command.
Tip: the most convenient way to write down clef is by using the autocomplete feature of the editor.
=1 ~1 (clef "treble")
=2 ~1 (clef "bass")
=3 ~1 (clef "percussion")
Instruments
Instruments can be set for every part using the instr command.
Example to define a grand piano: (instr "acoustic grand piano")
Tip: start writing something like pi.. and the autocomplete will propose all the piano instruments.
Pitches and rests
In Mirchord pitches are represented as single letters a-g while rests with the underscore character _
To define a major scale you can simply write: c d e f g a b

An example with rests:
=1 ~1
c8 _8 _4 _2 _1

Accidentals
Chromatic alterations are indicated by adding special symbols soon after the pitch letter (or pitch list in the chord case).
The # symbol is used for sharp/diesis.
The ampersand & symbol is used for flat/bemolle.
The § is the natural symbol (used for example to cancel the alteration in a particular key signature).
c# e&
produces:

Octaves
The octave in Mirchord can be specified in the current scope with the special ^ command followed by a number (scientific pitch notation)
c
in the ^4
octave is the middle C.
^5
states that the current octave in this scope is the fifth.
The octave calculated from the current scope can be raised or lowered using the ' and , marks respectively.
The octave in MirChord can be also specified as relative to the preceding pitch (a concept borrowed from the Lilypond relative mode) using the (octaveMode "relative")
command.
For example what follows is a C major scale that spans over two octaves
=1 ~1
(key "C" "maj")
(octaveMode "relative")
c d e f g a b c d e f g a b c

Example of octave marks:
=1 ~1
c c, c'

Durations
In the previous examples we have not specified the duration of notes so the default value of 1/4 is used.
There are two ways to specify the duration of a music element, i.e. note, chord, rest, ...
One option is the duration command: the symbol ` followed by a fraction
For example the directive `1/8
denotes a 1/8 duration (or 0.125 of a measure in decimal representation).
The other way to assign duration to a pitch or chord is to attach a number that represents the reciprocal of the actual duration to the pitch (or pitch list in case of a chord).
For dotted notes a . char should be placed after the duration number.
Using a special (durationMode "sticky")
command we can render the durations sticky, i.e. once defined in a scope (see below) they persist until the next change for all subsequent elements.
If a note duration does not fit within a measure length it is divided in more notes and ties are applied automatically between measure boundaries
=1 ~1
c2. e2 g2.

Ties
The tie symbol is the -
For example we can extend the normal duration of a whole note this way c- -c

Tuplets
Tuplets are defined with the tp command that takes as arguments a fraction and a variable number of notes.
The fraction indicates the number of actual notes over the number of notes usually filling the same space.
What follows is an example of a triplet:
=1 ~1
`1/8
(tp 3/2 g f e)

Phrases
A set of notes/chords enclosed in curly brackets { ... }
defines a phrase.
With a phrase it is possible to group a list of notes in a short motif with a dedicated scope (see below).
=1 ~1
{c e g}

Scopes
Scopes are "environments" that contain name bindings of contextual elements such as the current octave, duration, ecc... These elements are updated during code translation as explained above.
Scopes are created automatically for every voice of a staff and every defined phrase.
Chords
A chord (i.e. multiple pitches that sound at the same time) can be expressed by enclosing a list of pitches in squared brackets.
For example [c e g]2
is a C major chord with half measure duration.
While this is a straighforward route to manage chords, a more convoluted and elegant option is presented below.

Chord Symbols notation
A remarkable property of MirChord lies in its ability to define chords using the same syntactical structure usually found in lead sheets.
It is important to observe that in Mirchord notes are case sensitive for a reason: lowercase letters such as 'c' denote simple pitches while their uppercase countepart (ex. 'C') are reserved to chord symbols.
So the 'C' character is a C major chord.
The classic structure for a chord symbol construction is:
Root-Modifier-Extensions-(alterations)/BassNote (bass note is optional)
Some remarks on invertions:
If the pitch belongs to the chord than it denotes an invertion otherwise it is added as an additional note
the notation /BassNote indicates an inversion where the notes are moved an octave up while in the \BassNote case they are shifted an octave down.
Major chord first and second inversion
=1 ~1
C C\E C\G

A useful feature when using chords symbols is the /
char that allows to repeat the last typed chord.
Example of a chords progression
=1 ~1
`1/4 C / / / | Dm / / / | F / / / | G / / /

Other examples:
=1 ~1
[c e g]4 ^4 `1/4 C7 Cmaj7 Cmaj13(#11) ^5 `1/2 C `1/4 C/E C\E /

In the image above the (C root) chords depicted are respectively:
- Major chord using pitch list
- Dominant seventh chord
- Major seventh chord
- Major thirteenth chord (altered eleventh)
- Major chord
- Major chord with E bass octave up
- Major chord with E bass octave down
- Repeatition of the la last chord
Unpitched instruments
All the percussions should be placed in the same part (as channel 10 in MIDI). Different instruments are defined in different voices with the unpitched command.
The command indicates the instrument type and where, graphically, the elements should be rendered (pitch and octave).
For example the following code (unpitched "acousticbassdrum" "C" 4)
indicates that in the current voice all unpitched notes from now on are Acoustic Bass Drum rendered as ^4 c
Unpitched notes are represented with the lower letter x
or o
indifferently
Let's see an example with two different voices
=1
~1
(clef "percussion")
(unpitched "crashcymbala" "E" 5)
(durationMode "sticky")
x4 x x x x2 x2 x x8 x x x
~2
(unpitched "acousticbassdrum" "C" 4)
(durationMode "sticky")
x8 x4 x x8 x8 x4 x x8 x8 x4 x x8 x8 x4 x x8
~1 (* 3 {o16 o o o})

Universal Key/Scale
In MirChord notes and chord symbols can be expressed based on the current key signature.
For notes, solfeggio syllables can be used to represent the movable-do system.
For chords, Roman numerals can be used in place of capital letters.
=1 ~1
(key "B&" "maj")
I IV\I V\VII ^4 I
=2 ~1
(key "B&" "maj")
do re mi fa sol la si
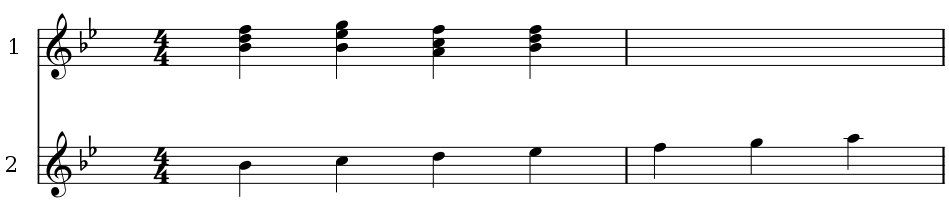
Examples
Fra Martino, the Italian version of the well known French nursery rhyme Frère Jacque
=1 ~1
(instr "flute")
(key "D" "min")
(durationMode "sticky") (octaveMode "relative")
^4 f4 g a f | f4 g a f | a4 b c2 | a4 b c2
^5 c8 d c b a4 f | ^5 c8 d c b a4 f
^4 f4 c f2 | f4 c f2
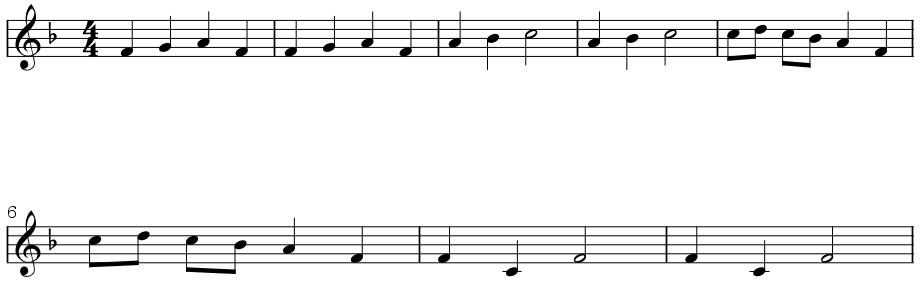
First measures from Invention N. 1 by Johann Sebastian Bach
=1 ~1
(key "C" "maj")
(clef "treble")
(name "Part 1")
(durationMode "sticky") (octaveMode "relative")
r16 c d e f d e c g'8 c b8 c | d16 g, a b c a b g d'8 g f g |
=2 ~1
(clef "bass")
(name "Part 2")
(durationMode "sticky") (octaveMode "relative")
^3 r2 r16 c d e f d e c | g'8 g, r4 r16 g a b c a b g |
=1
^5 e16 a g f e g f a g f e d c e d f | e d c b a c b d c b a g f# a g b
=2
^4 c8 b c d e g, a b | c e, f# g a b c4
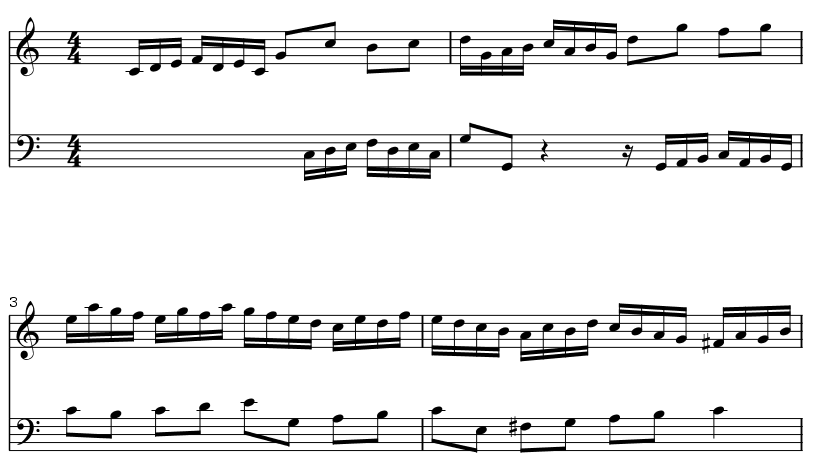
First measures from Marche by Carl Philipp Emanuel Bach
A Marche by Carl Philipp Emanuel Bach
=1 ~1
(instr "acoustic grand piano")
(key "E&" "maj")
(clef "treble")
(durationMode "sticky") (octaveMode "relative")
^4 _ _ _ e8 g | b4 c8 d e b g'4 | g8 f e d e4 [a, c] | [a c] [g b]2 [f a]4 | (tp 3/2 g8 f e) e2 a'4 |
=2 ~1
(key -3 "maj")
(clef "bass")
^3 _ _ _ e | g a8 f g4 e | b b' e, a | b2 b, | e4 g8 f g4 e | d b' b, b'
=1
(tp 3/2 f8 e d) d d d f e d
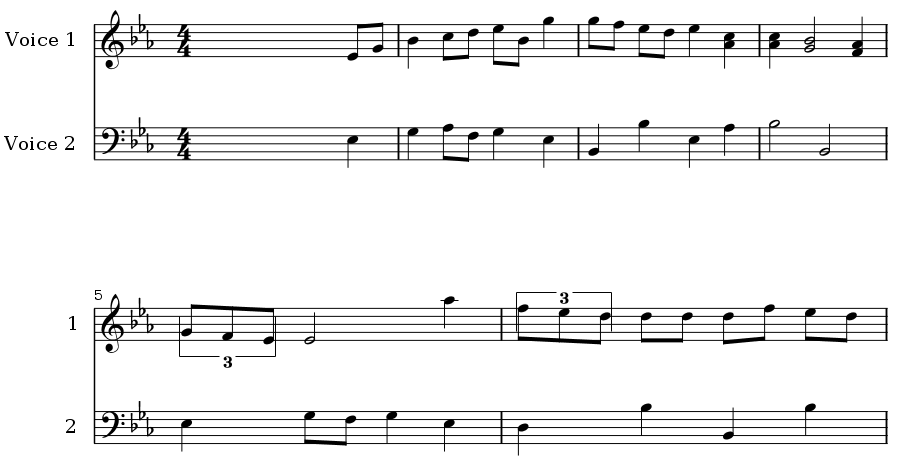
Symbols definition
The symbol definition is a language construct that specifies a name binding between an identifier and a phrase.
An identifier consists of a colon : followed by an alphanumeric string and after its definition (with the def command) can be called anywhere in the score.
For example valid symbols are :intro :chorus :ending
Symbols are similar to constants definition in programming languages and are tipically employed to reuse a particular motif (possibly transformed) several times.
=1 ~1
(def :mysymbol {c e g})
:mysymbol :mysymbol :mysymbol

Standard transformations
The interpreter by default ships with some built-in transformations often useful in music contexts.
As always the user is invited to make large use of the autocompletion feature of the editor in specifying transformations.
With the repetition command it is possible to duplicate a particular phrase.
For example the following code (* 3 {c e g2})
repeats the phrase 3 times.

An interesting tranformation is the zip function.
With this command it is possible to combine the pitches of the first phrase with the durations of the second phrase (a rhythmic pattern).
=1 ~1 (durationMode "sticky") (octaveMode "relative")
; equivalent to { ^5 c8 d c b a4 f }
(zip {^5 c d c b a f} {x8 x x x x4 x4})

Another common transformation allows to transpose every element of the phrase by a fixed number of semitones (half-steps):
=1 ~1 (durationMode "sticky") (octaveMode "relative")
(def :fra { ^4 f4 g a f })
:fra (transpose 12 :fra)

The following list of other conventional musical transformations have auto-explicative meaning and are built-in inside the framework:
- transpose
- transposeDiatonic
- invert
- invertDiatonic
- retrograde
- augment
- diminuition
Thanks to symbols definitions and the special commands that we have presented above we are now able to write a "smarter" and more synthetic version of Fra Martino
=1 ~1 (durationMode "sticky") (octaveMode "relative")
(instr "flute")
(key "D" "min")
(def :fra { ^4 f4 g a f }) ; Fra Martino campanaro
(def :dormi { ^4 a4 b c2 }) ; dormi tu?
(def :campane { ^5 c8 d c b a4 f }) ; suona le campane
(def :din { ^4 f4 c f2 }) ; din don dan
(* 2 :fra) (* 2 :dormi) (* 2 :campane) (* 2 :din)
that is rendered as above.Where to go next: Extending Mirchord with scripts.